Angular的controller as alias 语法解释
AngularJS 控制器在版本1.2 之后加入了某些改变,这次改变将会使程序结构更加明确,作用域范围更加清晰并且控制器更加灵活,建议使用this代替$scope
3 min read
By
myfreax
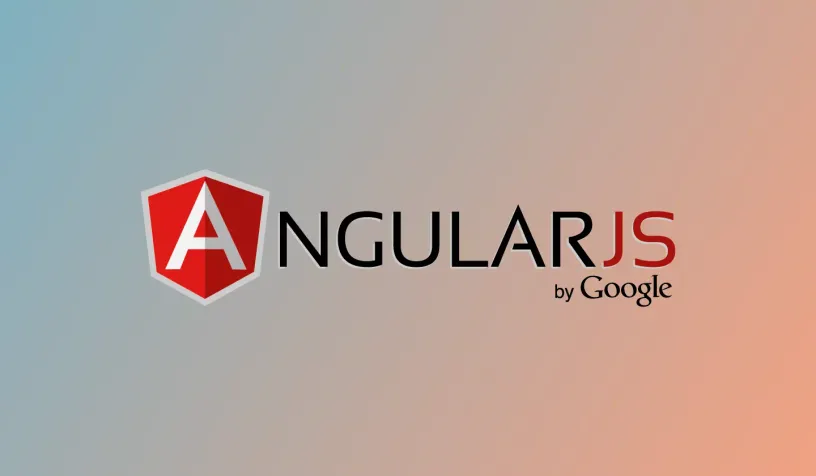
AngularJS 控制器在版本1.2 之后加入了某些改变,这次改变将会使程序结构更加明确,作用域范围更加清晰并且控制器更加灵活,建议使用this代替$scope
在1.2版本之前,控制器代码看起来像是这样的
// <div ng-controller="MainCtrl"></div>
app.controller('MainCtrl', function ($scope) {
$scope.title = 'Some title';
});
$scope从控制器中分离,我们需要依赖并且注入它,这通常不是很好的做法
app.controller('MainCtrl', function () {
this.title = 'Some title';
});
现在我们不需要注入$scope,但我们的代码会变得更加简洁
控制器当作类 Controllers as Classes
在javascript中 如果你想实例化一个类“class”,你需要这样做
var myClass = function () {
this.title = 'Class title';
}
var myInstance = new myClass();
通过myInstance
实例访问myClass
的方法和属性 ,在Angular我们获得实例是通过新的Controller as
语法并且快速声明和绑定
// we declare as usual, just using the `this` Object instead of `$scope`
app.controller('MainCtrl', function () {
this.title = 'Some title';
});
这是一个基本的类设置,当实例化控制器时,我们在DOM中获得实例赋值给变量
<div ng-controller="MainCtrl as main">
// MainCtrl doesn't exist, we get the `main` instance only MainCtrl 控制器是不存在的,我们仅仅获得main实例
</div>
this.title
在DOM中,我们需要通过实例访问它
<div ng-controller="MainCtrl as main">
{{ main.title }}
</div>
嵌套范围Nested scopes
<div ng-controller="MainCtrl">
{{ title }}
<div ng-controller="AnotherCtrl">
{{ title }}
<div ng-controller="YetAnotherCtrl">
{{ title }}
</div>
</div>
</div>
这样我们很有可能搞不清楚{{title}}
是来自那个控制器的,因为控制器嵌套意味下一级的控制器也会继承上一级控制器属性,这会让人感觉非常错乱,代码不易阅读,
<div ng-controller="MainCtrl as main">
{{ main.title }}
<div ng-controller="AnotherCtrl as another">
{{ another.title }}
<div ng-controller="YetAnotherCtrl as yet">
{{ yet.title }}
</div>
</div>
</div>
这样我们就会更加的清晰看到title是来自于那个控制器,下面我们看看使用parent scope 是怎么操作的
<div ng-controller="MainCtrl">
{{ title }}
<div ng-controller="AnotherCtrl">
Scope title: {{ title }}
Parent title: {{ $parent.title }}
<div ng-controller="YetAnotherCtrl">
{{ title }}
Parent title: {{ $parent.title }}
Parent parent title: {{ $parent.$parent.title }}
</div>
</div>
</div>
假如有更多的逻辑
<div ng-controller="MainCtrl as main">
{{ main.title }}
<div ng-controller="AnotherCtrl as another">
Scope title: {{ another.title }}
Parent title: {{ main.title }}
<div ng-controller="YetAnotherCtrl as yet">
Scope title: {{ yet.title }}
Parent title: {{ another.title }}
Parent parent title: {{ main.title }}
</div>
</div>
</div>