Python if..else语句
if控制语句是最基本,最著名的语句之一,用于根据特定条件执行代码。在本文中,我们将介绍Python if语句的基础
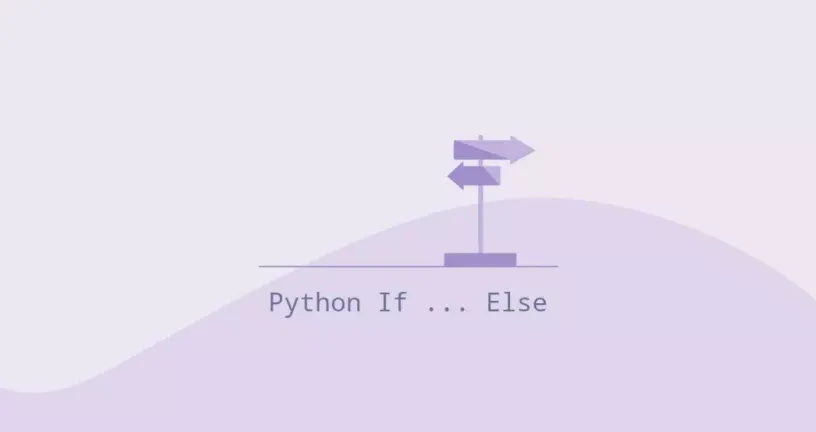
决策是计算机编程的最基本概念之一。 Python支持其他语言中常见的流程控制语句,并进行了一些修改。 if
控制语句是最基本,最著名的语句之一,用于根据特定条件执行代码。
在本文中,我们将介绍Python if
语句的基础。
Python if
语句
Python if
语句的最基本形式如下:
if EXPRESSION:
STATEMENT
if
语句以if
关键字开头,后跟条件表达式。
EXPRESSION
后必须跟(:
)冒号。 如果EXPRESSION
评估为True
,则执行STATEMENT
。 如果EXPRESSION
返回False
,则什么也没有发生,STATEMENT
被忽略。 STATEMENT
是任何语句,包括多个语句或进一步嵌套的if
语句。 要不执行任何语句,请使用pass
语句。
STATEMENT
块以缩进开始,以第一条未缩进的行结束。 大多数人选择使用4空格或者2空格缩进。 官方 Python代码样式指南建议每个缩进级别使用4个空格,并避免混合使用制表符和空格进行缩进。
让我们看一下以下示例脚本,该脚本检查给定数字是否大于5。
number = int(input('Enter a number: '))
if number > 5:
print(number, 'is greater than 5.')
将代码保存在文件中,然后从命令行运行它:
python test.py
脚本将提示您输入数字。 例如,如果输入10,则条件表达式的计算结果为True
(10大于5),并且将执行print
函数。
10 is greater than 5.
Python支持标准比较操作:
a == b
-如果a
和b
相等,则为true。a != b
-如果a
和b
不相等,则为true。a > b
-如果a
大于b
,则为true。a >= b
-如果a
等于或大于b
,则为true。a < b
-如果a
小于b
,则为true。a <= b
-如果a
等于或小于b
,则为true。
您还可以使用in
关键字来检查可迭代器(字符串,列表,元组,字典等)中是否存在该值:
s = 'myfreax'
if 'ze' in s:
print('True.')
以下是使用字典的另一个示例:
d = {'a': 2, 'b': 4}
if 'a' in d:
print('True.')
在字典上使用时,in
关键字检查字典是否具有特定的键。
要否定条件表达式,请使用逻辑not
运算符:
number = int(input('Enter a number: '))
if not number < 5:
print(number, 'is greater than 5.')
if..else表达式
if..else
语句计算条件,并根据结果执行两个语句之一。
Python if..else
陈述式采用以下形式:
if EXPRESSION:
STATEMENT1
else:
STATEMENT2
如果EXPRESSION
评估为True
,则将执行STATEMENT1
。 否则,如果EXPRESSION
返回False
,则将执行STATEMENT2
。 语句中只能有一个else
子句。
else
关键字必须以(:
)冒号结尾,并且与相应的if
关键字具有相同的缩进级别。
让我们在前面的示例脚本中添加else
子句:
number = int(input('Enter a number: '))
if number > 5:
print(number, 'is greater than 5.')
else:
print(number, 'is equal or less than 5.')
如果您运行代码并输入数字,脚本将根据数字是大于还是小于/等于5来打印不同的消息。
if..elif..else表达式
elif
关键字是else if
的缩写。
Python if..elif..else
采用以下形式:
if EXPRESSION1:
STATEMENT1
elif: EXPRESSION2:
STATEMENT2
else:
STATEMENT3
如果EXPRESSION1
评估为True
,则将执行STATEMENTS1
。 如果EXPRESSION2
评估为True
,则将执行STATEMENTS2
。 如果所有表达式的计算结果都不为True
,则执行STATEMENTS3
。
关键字elif
必须以(:
)冒号结尾,并且必须与相应的if
关键字处于相同的缩进级别。 语句中可以有一个或多个elif
子句。 else
子句是可选的。 如果不使用else
子句,并且所有表达式的求值为False
,则不会执行任何语句。
条件是按顺序评估的。 条件返回True
后,将不执行其余条件,并且程序控制移至if
语句的末尾。
让我们在之前的脚本中添加elif
子句:
number = int(input('Enter a number: '))
if number > 5:
print(number, 'is greater than 5.')
elif number < 5:
print(number, 'is less than 5.')
else:
print(number, 'is equal to 5.')
与大多数编程语言不同,Python没有switch
或case
语句。 多个elif
语句的序列可以替代switch
或 case
。
嵌套if
表达式
Python允许您在if
条语句中嵌套if
条语句。 通常,您应始终避免过度缩进,并尝试使用elif
而不是嵌套if
语句
以下脚本将提示您输入三个数字,并在数字中显示最大的数字。
number1 = int(input('Enter the first number: '))
number2 = int(input('Enter the second number: '))
number3 = int(input('Enter the third number: '))
if number1 > number2:
if number1 > number3:
print(number1, 'is the largest number.')
else:
print(number3, 'is the largest number.')
else:
if number2 > number3:
print(number2, 'is the largest number.')
else:
print(number3, 'is the largest number.')
这是输出的样子:
Enter the first number: 455
Enter the second number: 567
Enter the third number: 354
567 is the largest number.
多种条件
逻辑or
和and
运算符使您可以在if
语句中组合多个条件。
这是脚本的另一个版本,用于打印三个数字中最大的数字。 在此版本中,我们将使用逻辑and
运算符和elif
。而不是嵌套的if
语句。
number1 = int(input('Enter the first number: '))
number2 = int(input('Enter the second number: '))
number3 = int(input('Enter the third number: '))
if number1 > number2 and number1 > number3:
print(number1, 'is the largest number.')
elif number2 > number3 and number2 > number3:
print(number2, 'is the largest number.')
else:
print(number3, 'is the largest number.')
结论
if
,if..else
和if..elif..else
语句使您可以通过评估给定条件来控制Python执行的流程。如果您有任何问题或反馈,请随时发表评论。