Reflux的Todo示例
Reflux是一个简单的单向数据流应用程序架构库,其灵感来自Facebook的Flux状态管理库,相对来说Reflux会比Flux简单,我们可以从Reflux的Todo示例,来理解Reflux在实际的应用
2 min read
By
myfreax
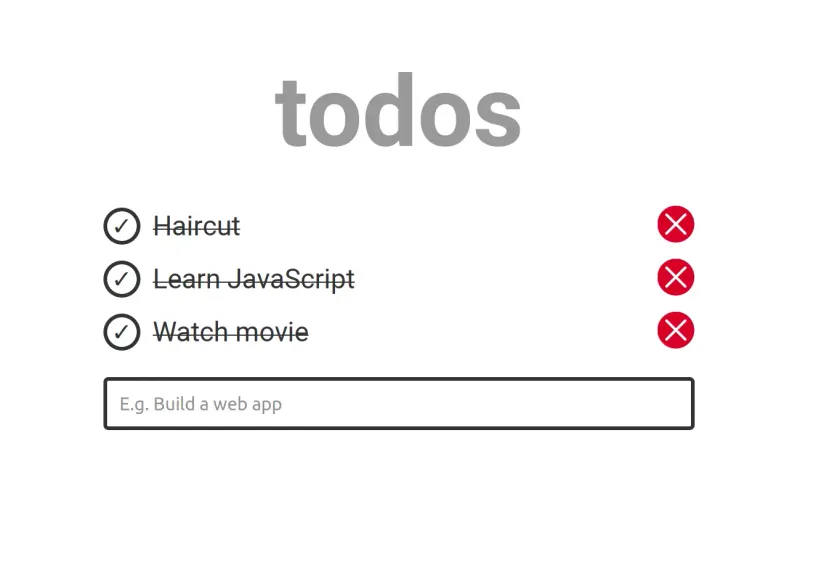
Reflux是一个简单的单向数据流应用程序架构库,其灵感来自Facebook的Flux状态管理库,相对来说Reflux会比Flux简单,我们可以从Reflux的Todo示例,来理解Reflux在实际的应用
store.js
/**
* Created by Freax on 2016/5/30.
* @website http://www.myfreax.com
*/
import Reflux from 'reflux'
import actions from './actions'
/**
* store 就像是react组件,也有生命周期
* 创建store可以使用createStore方法
*/
let store = Reflux.createStore({
listenables: [actions], //监听所有action
getInitialState:function () {
return this.list = [];
},
/**
* 监听action添加回调处理程序,
* 响应action操作
* User interaction -> component calls action -> store reacts and triggers -> components update
* store reacts and triggers
* @param text
*/
//注意回调方法命名规则action前面加上on并且把action第一个字母大写
onAddItem: function (text) {
this.list.push(text);
this.updateList(this.list);
},
updateList: function(list){
this.list = list;
//User interaction -> component calls action -> store reacts and triggers -> components update
this.trigger(list); //触发在上监听store变化的组件更新 components update
//store triggers -> components update
}
});
export default store;
actions.js
/**
* Created by Freax on 2016/5/30.
* @website http://www.myfreax.com
*/
import Reflux from 'reflux'
/**
* 每个action就像是一个特定的事件通道,action由component来回调
* store监听着全部action,component监听store的变化
* 可以看出数据流是这样的 用户交互 -> component回调action -> store响应并且触发 -> components更新
*
*/
export default Reflux.createActions([
'addItem'
]);
Items.js
/**
* Created by Freax on 2016/5/30.
* @website http://www.myfreax.com
*/
import React from 'react'
import Item from './Item'
import Reflux from 'reflux'
import store from './store'
export default React.createClass({
//当 store trigger(updatedlist)时,将会 setState({list:updatedlist})更新组件
mixins:[Reflux.connect(store,'list')],
render(){
let items = this.state.list.map((value)=> {
return <Item key={value}>{value}</Item>
});
return (
<div>
<ul className="collection" style={{display:items.length?'block':'none'}}>
{items?items:''}
</ul>
</div>
);
}
});
完整代码Github