JavaScript 重定向
您将学习如何使用 JavaScript 重定向到新的 URL 或页面
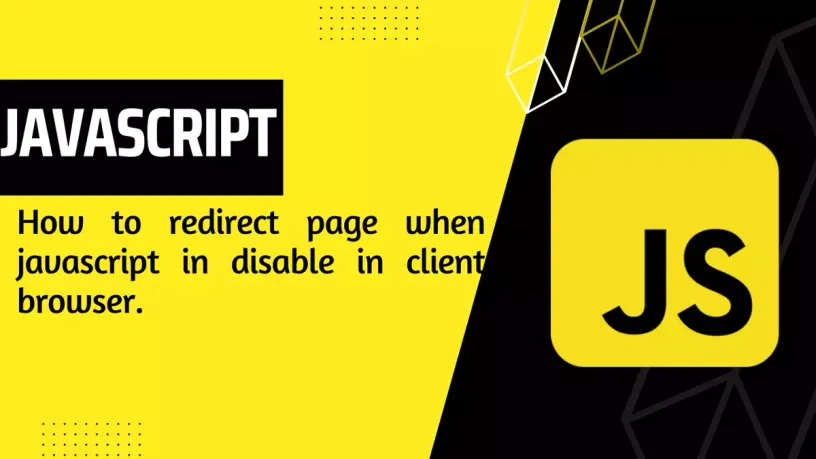
在本教程中,您将学习如何使用 JavaScript 重定向到新的 URL 或页面。
JavaScript 重定向简介
有时,您希望将用户从一个页面重定向到具有不同 URL 的另一页面。
例如,您可以创建一个应用程序登录页面,将用户重定向到 Google Play 或 Apple Store,具体取决于他们的智能手机所使用的操作系统。
因此,您需要检测智能手机的操作系统。如果操作系统是 IOS,则重定向至 Apple Store。如果操作系统是 Android,则重定向至 Google Play。
可以使用 JavaScript 重定向 API 在 Web 浏览器中完成重定向。值得注意的是,JavaScript 重定向完全在 Web 浏览器运行。
因此,它不会像服务器重定向那样返回状态代码 301。如果您将站点移动到单独的域或为旧页面创建新 URL,则应始终使用服务器重定向。
重定向到新的 URL
要将 Web 浏览器从当前页面重定向到新 URL 到新页面,请使用 location
对象:
location.href = 'new_url';
例如:
location.href = 'https://www.myfreax.com/';
为 location
对象的 href
属性赋值与调用 location
对象的 assign()
方法具有相同的效果:
location.assign('https://www.myfreax.com/');
这些调用都会重定向到新的 URL 并在浏览器的历史堆栈中创建一条记录。这意味着您可以通过浏览器的后退按钮返回到上一页。
要重定向到新 URL 而不在浏览器的历史堆栈中创建新的记录,请使用 location
对象的 replace()
方法:
location.replace('https://www.myfreax.com/');
以下示例创建一个页面,如果操作系统是 Android 或 IOS,则将 Web 浏览器重定向到 Google Play 或 Apple Store。否则,它将显示一条消息,指示操作系统不受支持:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Redirect</title>
</head>
<body>
<div class="message"></div>
<script src="js/app.js"></script>
</body>
</html>
js/app.js
const apps = {
Android: 'https://play.google.com/',
iOS: 'https://www.apple.com/store',
};
const platform = () => {
let userAgent = navigator.userAgent || navigator.vendor || window.opera;
if (/windows phone/i.test(userAgent)) return 'Windows Phone';
if (/android/i.test(userAgent)) return 'Android';
if (/iPad|iPhone|iPod/.test(userAgent) && !window.MSStream) return 'iOS';
return null;
};
const redirect = () => {
let os = platform();
if (os in apps) {
location.replace(apps[os]);
} else {
const message = document.querySelector('.message');
message.innerText = 'Your OS is not supported';
}
};
redirect();
代码是如何运行的。
首先,定义一个 apps
对象,键为 OS,值为 Google Play 和 Apple Store 的 URL:
const apps = {
Android: 'https://play.google.com/',
iOS: 'https://www.apple.com/store',
};
其次,定义一个检测操作系统的函数:
const platform = () => {
let userAgent = navigator.userAgent || navigator.vendor || window.opera;
if (/windows phone/i.test(userAgent)) return 'Windows Phone';
if (/android/i.test(userAgent)) return 'Android';
if (/iPad|iPhone|iPod/.test(userAgent) && !window.MSStream) return 'iOS';
return null;
};
javascripttutorial
第三,创建检测操作系统并根据检测到的操作系统重定向 Web 浏览器的函数 redirect()
:
const redirect = () => {
let os = platform();
if (os in apps) {
location.replace(apps[os]);
} else {
const message = document.querySelector('.message');
message.innerText = 'Your OS is not supported';
}
};
最后,调用 redirect()
函数:
redirect();
重定向到相对 URL
下面脚本重定向到与 about.html
当前页面处于同一级别的页面。
location.href = 'about.html';
下面脚本重定向到位于 root
文件夹的 contact.html
页面:
location.href = '/contact.html';
页面加载时重定向
如果您想在加载时重定向到新页面,请使用以下代码:
window.onload = function() {
location.href = "https://www.myfreax.com/";
}
结论
要重定向到新的 URL 或页面,请将新 URL 分配给属性 location.href
或使用 location.assign()
方法。
location.replace()
方法会重定向到新的 URL,但不会在浏览器的历史堆栈中创建条目。