Python Tuples元组
Tuples类似于列表,主要区别在于,列表是可变的,而Tuples是不可变的。 这意味着创建后不能改变Tuples元组
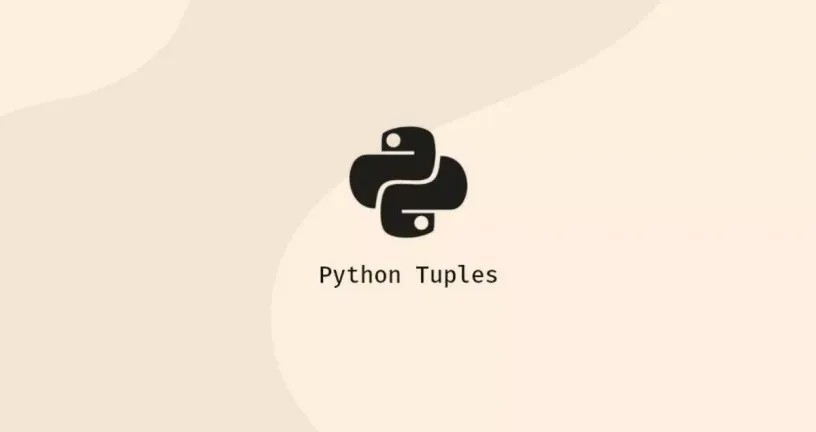
Python有几种顺序数据类型,允许您以有组织和有效的方式存储数据集合。 基本序列类型是字符串,列表,元组和范围对象。
本文将介绍Python Tuples元组的基础知识。 我们将向您展示如何创建Tuples元组,访问元素,解压缩Tuples元组等。
Tuples类似于列表,主要区别在于,列表是可变的,而Tuples是不可变的。 这意味着创建后不能改变Tuples元组。
Tuples元组可以存储异构和均匀的数据,但通常用于存储异质元素的集合。
创建元组
通过将元素放置在一对圆形括号[]
中,由逗号分隔来创建Tuples元组。 它们可以有任何数量的元素,可以是不同类型的元素。 这是一个例子:
colors = ('orange', 'white', 'green')
Tuples元组可以具有混合数据类型的元素。 您还可以声明嵌套Tuples元组,元素可以是列表,Tuples元组或词典:
my_tuple = (1, False, ["red", "blue"], ("foo", "bar"))
它们之间没有元素的圆形括号表示空元组:
my_tuple = ()
以创建只有一个元素的Tuples元组,您必须在元素之后添加逗号:
my_tuple = (1)
type(my_tuple)
my_tuple = (1,)
type(my_tuple)
<class 'int'>
<class 'tuple'>
可以使用tuple()
构造函数构建Tuples元组:
colors_list = ['orange', 'white', 'green']
colors_typle = tuple(colors_list)
print(type(colors_typle))
<class 'tuple'>
构造Tuples元组的另一种方法是使用Tuples元组打包功能,允许您从逗号分隔的对象序列创建元组:
directions = "North", "South", "East", "West"
print(type(directions))
<class 'tuple'>
访问元组元素
元组项目可以通过其索引引用。 索引是整数,并从0
到n-1
,其中n
是项目的数量:
my_tuple = ["a", "b", "c", "d"]
0 1 2 3
在Python索引中使用方括号指定:
my_tuple[index]
例如访问元组的第三个元素,您将tuple_name[2]
:
directions = ("North", "South", "East", "West")
directions[2]
'East'
如果引用一个不存在的索引,则提出了IndexError
异常:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: tuple index out of range
访问嵌套元组中的项目使用多个索引:
my_tuple = (1, False, ["red", "blue"], ("foo", "bar"))
my_tuple[3][1]
'bar'
您还可以使用负索引访问元组元素。 最后一项称为-1
,第二项作为-2
等:
my_tuple = ("a", "b", "c", "d")
-4 -3 -2 -1
directions = ("North", "South", "East", "West")
directions[-2]
'East'
切片Tuples元组
在Python中,您可以使用以下形式切片和其他顺序数据类型:
sequence[start:stop:step]
start
是提取开始的指标。 当使用否定索引时,它表示从元组末端的偏移量。 如果省略此参数,则切片从索引0开始0.stop
是最终提取之前的指数; 结果不包括“停止”元素。 当使用否定索引时,它表示从元组末端的偏移量。 如果省略此参数或大于元组长的长度,则切片转到元组的末尾。step
是一个可选的参数,并指定切片的步骤。 未指定时,它默认为1.如果使用负值,则切片以相反的顺序接受元素。
切片元组的结果是包含提取元素的新元组。
以下表格在Python中是合法的:
T[:] # copy whole tuple
T[start:] # slice the tuple starting from the element with index "start" to the end of the tuple.
T[:stop] # slice the tuple starting from the begging up to but not including the element with index "stop".
T[start:stop] # slice the tuple starting from the element with index "start" up to but not including the element with index "stop".
stop"
T[::step] # slice the tuple with a stride of "step"
下面是如何将元组切片从具有索引1的元素切割,但不包括具有索引4的元素:
vegetables = ('Potatoes', 'Garlic', 'Celery', 'Carrots', 'Broccoli')
vegetables[1:4]
('Garlic', 'Celery', 'Carrots')
打开包装元组
在Python功能中打开包装的序列,允许您将序列对象带到变量。 以下是一个例子:
colors = ('orange', 'white', 'green')
a, b, c = colors
print(a)
print(b)
print(c)
根据其位置的元组元素的值被分配给左侧的变量:
orange
white
green
在解包组合时,左侧元组上的变量的数量必须与元组元素的数量相同。 否则,您将获得ValueError
异常。
colors = ('orange', 'white', 'green')
a, b = colors
ValueError: too many values to unpack (expected 2)
当方法或函数返回一系列对象时,解压缩是方便的:
def square_area_circumference(side_lenght):
return side_lenght * side_lenght, side_lenght * 4
area, circumference = square_area_circumference(5)
print(area)
print(circumference)
25
20
改变元组
由于元组是不可变数据结构,因此我们无法直接更新它们。 您无法添加,更改,删除元组中的元素。
如果您尝试更改元组元素,则会收到TypeError
异常:
colors = ("orange", "white", "green")
colors[1] = "blue"
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
可以改变可变元组项目的元素。 例如,如果元组有列表作为其元素之一,则可以更新列表元素:
my_tuple = (1, 2, [5, 6, 7])
my_tuple[2][1] = 4
print(my_tuple)
(1, 2, [5, 4, 7])
元组长
内置len()
函数返回给定对象的总项目数。
找到一个元组长,将其作为参数传递给len()
功能:
len(L)
以下是一个例子:
colors = ("orange", "white", "green")
lenght = len(colors)
print(lenght)
3
通过元组迭代
迭代元组中的所有元素,可以使用 for
循环:
directions = ("North", "South", "East", "West")
for direction in directions:
print(direction)
North
South
East
West
如果您需要索引,您可以使用多种方法。 最常见的方式是组合 range()
和len()
函数或使用内置 enumerate()
功能。
下面的示例显示了如何检索元组中每个项目的索引和值:
directions = ("North", "South", "East", "West")
for i in range(len(directions)):
print("Index {} : Value {}".format(i, directions[i]))
Index 0 : Value North
Index 1 : Value South
Index 2 : Value East
Index 3 : Value West
而不是使用range(len(...))
模式,您可以使用enumerate()
函数以更粘合的方式循环组元组:
directions = ("North", "South", "East", "West")
for index, value in enumerate(directions):
print("Index {} : Value {}".format(index, value))
Index 0 : Value North
Index 1 : Value South
Index 2 : Value East
Index 3 : Value West
检查元素是否存在
检查元组中是否存在元素,可以使用in
和not in
运算符:
colors = ("orange", "white", "green")
print("orange" in colors)
输出将是True
或False
:
True
这里是另一个例子,使用 if
陈述:
colors = ("orange", "white", "green")
if "blue" not in colors:
print("no")
else:
print("yes")
no
元组方法
元组对象接受以下方法:
count(x)
- 返回元组中出现的次数“x”。index(x)
- 返回具有“X”值的第一次出现元素的位置。
以下是一个简单的示例,展示了如何使用这些方法:
my_tuple = ("a", "s", "s", "q", "a", "n")
print(my_tuple.count('a'))
print(my_tuple.index('a'))
2
0
结论
在Python中,元组是一旦创建时无法更改的对象的不可变序列。
如果您有任何问题或反馈,请随时留下评论。